This post explores some of PowerShell commands that I’ve found invaluable for rapid triage during investigations.
Process Management
1
| Stop-Process -Name "NameOfProcess" -Force
|
- List Running Processes, Parent Process, and Path:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| Get-WmiObject -Class Win32_Process | ForEach-Object {
$processID = $_.ProcessID
$parentProcessID = $_.ParentProcessID
$parentProcess = Get-WmiObject -Class Win32_Process -Filter "ProcessID='$parentProcessID'"
[PSCustomObject]@{
ProcessName = $_.Name
ProcessID = $processID
ParentProcessName = $parentProcess.Name
ExecutablePath = $_.ExecutablePath
CommandLine = $_.CommandLine
}
}
|
- Generate Hashes of All Running Processes:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
| Get-Process | ForEach-Object {
try {
$hash = Get-FileHash $_.Path -Algorithm SHA256
Write-Output "$($_.ProcessName), $hash"
}
catch {
Write-Output "$($_.ProcessName), $_.Exception.Message"
}
}
|
1
| Get-NetTCPConnection -State Listen | Select-Object LocalAddress, LocalPort, OwningProcess, OwningProcessName
|
1
| Get-NetNeighbor | Where-Object {$_.State -eq 'Reachable'} | Format-Table IPAddress, LinkLayerAddress, State
|
- Check for Open RDP Sessions
1
| Get-WmiObject -Class Win32_Session | Where-Object {$_.SessionType -eq "RDP"}
|
- Check DNS Cache for Signs of DNS Hijacking
- Get Active Network Shares
File System and Registry
1
2
|
Get-ChildItem [file] | Format-List *
|
1
2
|
Get-ItemProperty C:\Test\Weather.xls | Format-List
|
1
2
|
Get-FileHash -Algorithm MD5 C:\path\to\file
|
1
2
|
gci -r | sort -descending -property length | select -first 10 name, length, directory
|
- Files Created or Modified Between Two Dates:
1
2
|
Get-ChildItem -Path "C:\Path\to\folder" -Recurse | Where-Object {($_.CreationTime -ge (Get-Date "2023-06-11")) -and ($_.CreationTime -le (Get-Date "2023-06-22")) -or ($_.LastWriteTime -ge (Get-Date "2023-06-11")) -and ($_.LastWriteTime -le (Get-Date "2023-06-22"))} | Select-Object FullName, CreationTime, LastWriteTime
|
1
2
|
Get-ChildItem -Path C:\ -Recurse -File | Get-FileHash | Where-Object Hash -eq 'HASH_VALUE' | Select-Object Path
|
1
2
|
Get-ChildItem -Path "C:\Path\To\Search" -Filter "FileName"
|
- File Changed in Last 24 Hours:
1
2
|
Get-ChildItem -Path C:\ -Recurse -File -Force | Where-Object { $_.LastWriteTime -ge (Get-Date).AddHours(-6) }
|
- Files Created in Last 24 Hours:
1
2
|
Get-ChildItem -Path C:\ -Recurse -File -Force | Where-Object { $_.CreationTime -ge (Get-Date).AddHours(-6) }
|
- Obtain List of All Files:
1
2
3
4
|
tree C:\ /F > output.txt
dir C:\ /A:H /-C /Q /R /S /X
|
1
2
|
Remove-Item -Path "HKLM:\Path\To\Registry\Key" -Recurse
|
1
2
|
Get-ChildItem -Path 'HKLM:\SOFTWARE\Policies\Microsoft\Windows\WindowsUpdate\'
|
- Retrieve Auto-Start Locations (Startup Programs)
1
| Get-WmiObject -Query "SELECT * FROM Win32_StartupCommand" | Select-Object Name, Command, User
|
1
| Get-ItemProperty HKLM:\Software\Microsoft\Windows\CurrentVersion\Uninstall\* | Select-Object DisplayName, DisplayVersion, InstallDate
|
Event Logs
1
2
|
Get-WinEvent -FilterHashtable @{logname="Microsoft-Windows-Sysmon/Operational"; id=1} | Where-Object {$_.Properties[20].Value -like "*rdpclip*"} | fl
|
1
2
|
Get-WinEvent -FilterHashtable @{LogName='Security';ID=4624,4625} | Select-Object TimeCreated, Id, Message
|
- Search Event Logs by Keyword:
1
2
|
Get-WinEvent -FilterHashtable @{ LogName='Security';} | Select Timecreated,LogName,Message | Where {$_.Message -like "*test*"} | FL
|
1
2
|
Get-WinEvent -Path .\Microsoft-Windows-Sysmon%4Operational.evtx | Export-CSV foo.csv
|
1
2
|
Get-LocalUser | Select *
|
- Get the SID from a Given Username:
1
2
3
4
5
6
7
8
|
$username = "Domain\Username"
$account = New-Object System.Security.Principal.NTAccount($username)
$sid = $account.Translate([System.Security.Principal.SecurityIdentifier]).Value
Write-Output $sid
|
1
2
3
4
5
6
7
8
|
$SID = "S-1-5-21-329068152-1454471165-1417001333-12984448"
$account = New-Object System.Security.Principal.SecurityIdentifier($SID)
$username = $account.Translate([System.Security.Principal.NTAccount]).Value
Write-Output $username
|
Job and Task Management
1
2
|
Get-ScheduledTask | Where-Object { $_.State -eq 'Ready' }
|
File Operations
1
2
|
Copy-Item -Source \\server\share\test -Destination C:\path\
|
- Download File from Internet:
1
2
|
Invoke-WebRequest -Uri "https://download.sysinternals.com/files/Sysmon.zip" -OutFile $env:TEMP\sysmon.zip
|
1
2
|
Expand-Archive -Path <SourcePathofZipFile> -DestinationPath <DestinationPath>
|
1
2
|
Read-Host -Prompt "Enter the encryption passphrase" -AsSecureString | ConvertFrom-SecureString | Set-Content -Path "C:\Path\EncryptedFile.txt"
|
Web Operations
1
2
3
4
5
6
|
$filePath = "C:\Path\To\File.txt"
$uploadUrl = "http://example.com/upload"
Invoke-RestMethod -Uri $uploadUrl -Method POST -InFile $filePath
|
Miscellaneous
- Generate Random Data (10MB):
1
2
|
$fileSizeInMB = 10; $filePath = "$PWD\file.txt"; $data = [byte[]]::new($fileSizeInMB * 1MB); $random = New-Object -TypeName System.Random; $random.NextBytes($data); [System.IO.File]::WriteAllBytes($filePath, $data)
|
- Regex Matching for Specific String (“feras”):
1
2
|
Select-String -Path C:\path\strings.txt -Pattern '\bferas\b' -AllMatches | % { $_.Matches } | % { $_.Value }
|
Linux cut command
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| Cut function powershell
function cut {
param(
[Parameter(ValueFromPipeline=$True)] [string]$inputobject,
[string]$delimiter='\s+',
[string[]]$field
)
process {
if ($field -eq $null) { $inputobject -split $delimiter } else {
($inputobject -split $delimiter)[$field] }
}
}
|
PowerShell with Security Utilities
PowerShell enhances security utilities like Sysinternals for effective IR:
Sysinternals: Start utilities like Process Explorer:
1
| Start-Process "C:\Path\To\ProcessExplorer.exe"
|
Remote Commands: Use PsExec to run commands on target machines:
1
| .\PsExec.exe \\TargetMachine cmd
|
Data Collection: Retrieve logs from Sysmon:
1
| Get-WinEvent -LogName 'Microsoft-Windows-Sysmon/Operational'
|
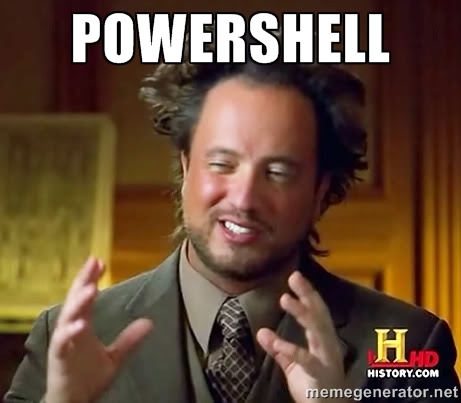